|
In the previous chapter, you drew squares with side lengths that were firmly implemented in the program. There may also be times when you want to enter the side length with an input dialog. In order to do this, the program needs to store the entered number as a variable. You can see the variable as a container, the content of which you can access with a name. So, in short, a variable has a name and a value. You can freely choose the name of a variable, but they cannot be keywords or names with special characters. Moreover the name cannot start with a number.
With the notation a = 2 you create the container that you can access with the name a and put the number 2 inside. In the future we will say that you are defining a variable a and assigning a value to it.
|
|
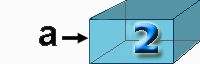
a = 2 : variable definition (assignment)
|
You can only put one object into the container. Later in your program, when you want to store another number 3 under the name a, write a = 3 [more...In Python are also numbers objects. a is a reference variable
and a new assignment creates a new object. The old object is removed by the Garbage Collector.]. |
|
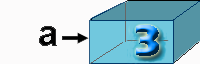
a = 3 : new assignment
|
So then what happens when you write a = a + 5? You take the number that is currently in the container, accessed with the name a, and therefore the number 3 is added to the number 5. The result adds up to 8 and it is again saved under the name a.
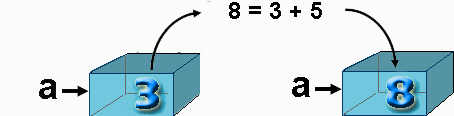
Therefore, the equal sign does not mean the same thing in computer programming as it does in mathematics. It does not define an equation, but rather a variable definition or an assignment
[more...In some programming languages are used therefore for assigning a different notation, for example, : = or MAKE].
PROGRAMMING CONCEPTS:
Variable definition, assignment |